注意
转到结尾 下载完整的示例代码。或通过 JupyterLite 或 Binder 在浏览器中运行此示例
稳健协方差估计和马氏距离相关性#
此示例演示了在高斯分布数据上使用马氏距离进行协方差估计。
对于高斯分布数据,可以使用观测值 \(x_i\) 的马氏距离计算其到分布模式的距离
其中 \(\mu\) 和 \(\Sigma\) 是基础高斯分布的位置和协方差。
在实践中,\(\mu\) 和 \(\Sigma\) 被一些估计值代替。标准协方差最大似然估计 (MLE) 对数据集中异常值的存在非常敏感,因此下游马氏距离也是如此。最好使用稳健的协方差估计器来保证估计对数据集中的“错误”观测值具有抵抗力,并且计算出的马氏距离准确地反映了观测值的真实组织。
最小协方差行列式估计器 (MCD) 是一种稳健的、高击穿点 (即它可以用来估计高度污染的数据集的协方差矩阵,最多可达 \(\frac{n_\text{samples}-n_\text{features}-1}{2}\) 个异常值) 协方差估计器。MCD 背后的思想是找到 \(\frac{n_\text{samples}+n_\text{features}+1}{2}\) 个观测值,其经验协方差具有最小的行列式,从而产生一个“纯净”的观测值子集,从中可以计算位置和协方差的标准估计值。MCD 由 P.J.Rousseuw 在 [1] 中提出。
此示例说明了马氏距离如何受到异常数据的影响。当使用基于标准协方差 MLE 的马氏距离时,来自污染分布的观测值与来自真实高斯分布的观测值无法区分。使用基于 MCD 的马氏距离,这两个总体变得可以区分。相关的应用包括异常值检测、观测值排序和聚类。
注意
参考文献
# Authors: The scikit-learn developers
# SPDX-License-Identifier: BSD-3-Clause
生成数据#
首先,我们生成一个包含 125 个样本和 2 个特征的数据集。这两个特征都服从高斯分布,均值为 0,但特征 1 的标准差为 2,特征 2 的标准差为 1。接下来,用 25 个样本的高斯异常值样本替换 25 个样本,其中特征 1 的标准差为 1,特征 2 的标准差为 7。
import numpy as np
# for consistent results
np.random.seed(7)
n_samples = 125
n_outliers = 25
n_features = 2
# generate Gaussian data of shape (125, 2)
gen_cov = np.eye(n_features)
gen_cov[0, 0] = 2.0
X = np.dot(np.random.randn(n_samples, n_features), gen_cov)
# add some outliers
outliers_cov = np.eye(n_features)
outliers_cov[np.arange(1, n_features), np.arange(1, n_features)] = 7.0
X[-n_outliers:] = np.dot(np.random.randn(n_outliers, n_features), outliers_cov)
结果比较#
下面,我们将基于 MCD 和 MLE 的协方差估计器拟合到我们的数据中,并打印估计的协方差矩阵。请注意,使用基于 MLE 的估计器 (7.5) 估计的特征 2 的方差远高于基于 MCD 的稳健估计器 (1.2)。这表明基于 MCD 的稳健估计器对异常值样本的抵抗力强得多,这些异常值样本的设计目的是在特征 2 中具有更大的方差。
import matplotlib.pyplot as plt
from sklearn.covariance import EmpiricalCovariance, MinCovDet
# fit a MCD robust estimator to data
robust_cov = MinCovDet().fit(X)
# fit a MLE estimator to data
emp_cov = EmpiricalCovariance().fit(X)
print(
"Estimated covariance matrix:\nMCD (Robust):\n{}\nMLE:\n{}".format(
robust_cov.covariance_, emp_cov.covariance_
)
)
Estimated covariance matrix:
MCD (Robust):
[[ 3.26253567e+00 -3.06695631e-03]
[-3.06695631e-03 1.22747343e+00]]
MLE:
[[ 3.23773583 -0.24640578]
[-0.24640578 7.51963999]]
为了更好地可视化差异,我们绘制了两种方法计算的马氏距离的等高线。请注意,基于稳健 MCD 的马氏距离更适合内点(黑色点),而基于 MLE 的距离受异常点(红色点)的影响更大。
import matplotlib.lines as mlines
fig, ax = plt.subplots(figsize=(10, 5))
# Plot data set
inlier_plot = ax.scatter(X[:, 0], X[:, 1], color="black", label="inliers")
outlier_plot = ax.scatter(
X[:, 0][-n_outliers:], X[:, 1][-n_outliers:], color="red", label="outliers"
)
ax.set_xlim(ax.get_xlim()[0], 10.0)
ax.set_title("Mahalanobis distances of a contaminated data set")
# Create meshgrid of feature 1 and feature 2 values
xx, yy = np.meshgrid(
np.linspace(plt.xlim()[0], plt.xlim()[1], 100),
np.linspace(plt.ylim()[0], plt.ylim()[1], 100),
)
zz = np.c_[xx.ravel(), yy.ravel()]
# Calculate the MLE based Mahalanobis distances of the meshgrid
mahal_emp_cov = emp_cov.mahalanobis(zz)
mahal_emp_cov = mahal_emp_cov.reshape(xx.shape)
emp_cov_contour = plt.contour(
xx, yy, np.sqrt(mahal_emp_cov), cmap=plt.cm.PuBu_r, linestyles="dashed"
)
# Calculate the MCD based Mahalanobis distances
mahal_robust_cov = robust_cov.mahalanobis(zz)
mahal_robust_cov = mahal_robust_cov.reshape(xx.shape)
robust_contour = ax.contour(
xx, yy, np.sqrt(mahal_robust_cov), cmap=plt.cm.YlOrBr_r, linestyles="dotted"
)
# Add legend
ax.legend(
[
mlines.Line2D([], [], color="tab:blue", linestyle="dashed"),
mlines.Line2D([], [], color="tab:orange", linestyle="dotted"),
inlier_plot,
outlier_plot,
],
["MLE dist", "MCD dist", "inliers", "outliers"],
loc="upper right",
borderaxespad=0,
)
plt.show()
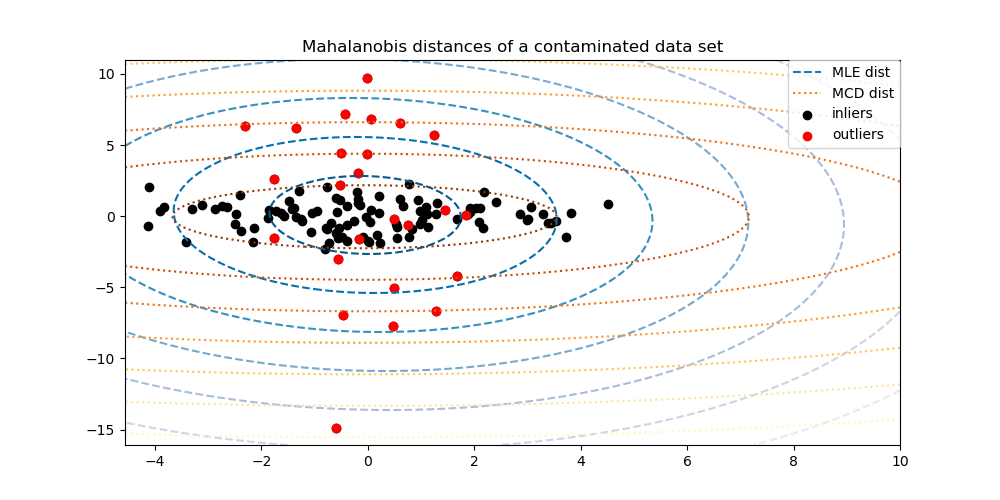
最后,我们强调了基于 MCD 的马氏距离区分异常值的能力。我们取马氏距离的立方根,得到近似正态分布(如 Wilson 和 Hilferty 所建议的 [2]),然后用箱线图绘制内点和异常值样本的值。对于基于稳健 MCD 的马氏距离,异常值样本的分布与内点样本的分布更加分离。
fig, (ax1, ax2) = plt.subplots(1, 2)
plt.subplots_adjust(wspace=0.6)
# Calculate cubic root of MLE Mahalanobis distances for samples
emp_mahal = emp_cov.mahalanobis(X - np.mean(X, 0)) ** (0.33)
# Plot boxplots
ax1.boxplot([emp_mahal[:-n_outliers], emp_mahal[-n_outliers:]], widths=0.25)
# Plot individual samples
ax1.plot(
np.full(n_samples - n_outliers, 1.26),
emp_mahal[:-n_outliers],
"+k",
markeredgewidth=1,
)
ax1.plot(np.full(n_outliers, 2.26), emp_mahal[-n_outliers:], "+k", markeredgewidth=1)
ax1.axes.set_xticklabels(("inliers", "outliers"), size=15)
ax1.set_ylabel(r"$\sqrt[3]{\rm{(Mahal. dist.)}}$", size=16)
ax1.set_title("Using non-robust estimates\n(Maximum Likelihood)")
# Calculate cubic root of MCD Mahalanobis distances for samples
robust_mahal = robust_cov.mahalanobis(X - robust_cov.location_) ** (0.33)
# Plot boxplots
ax2.boxplot([robust_mahal[:-n_outliers], robust_mahal[-n_outliers:]], widths=0.25)
# Plot individual samples
ax2.plot(
np.full(n_samples - n_outliers, 1.26),
robust_mahal[:-n_outliers],
"+k",
markeredgewidth=1,
)
ax2.plot(np.full(n_outliers, 2.26), robust_mahal[-n_outliers:], "+k", markeredgewidth=1)
ax2.axes.set_xticklabels(("inliers", "outliers"), size=15)
ax2.set_ylabel(r"$\sqrt[3]{\rm{(Mahal. dist.)}}$", size=16)
ax2.set_title("Using robust estimates\n(Minimum Covariance Determinant)")
plt.show()
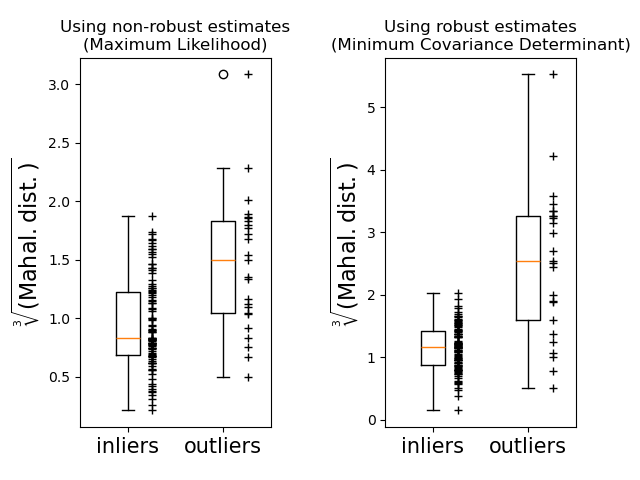
脚本总运行时间:(0 分钟 0.277 秒)
相关示例