注意
点击此处下载完整示例代码,或通过JupyterLite或Binder在浏览器中运行此示例。
使用多项式核近似进行可扩展学习#
此示例说明了如何使用PolynomialCountSketch
高效地生成多项式核特征空间近似值。这用于训练线性分类器,以逼近核化分类器的精度。
我们使用Covtype数据集[2],尝试重现Tensor Sketch原始论文[1]中的实验,即PolynomialCountSketch
实现的算法。
首先,我们计算线性分类器在原始特征上的精度。然后,我们使用PolynomialCountSketch
生成的各种数量的特征(n_components
)训练线性分类器,以可扩展的方式逼近核化分类器的精度。
# Authors: The scikit-learn developers
# SPDX-License-Identifier: BSD-3-Clause
准备数据#
加载Covtype数据集,该数据集包含581,012个样本,每个样本具有54个特征,分布在6个类别中。该数据集的目标是仅根据制图变量预测森林覆盖类型(没有遥感数据)。加载后,我们将它转换为二元分类问题,以匹配LIBSVM网页[2]中数据集的版本,该版本在[1]中使用。
from sklearn.datasets import fetch_covtype
X, y = fetch_covtype(return_X_y=True)
y[y != 2] = 0
y[y == 2] = 1 # We will try to separate class 2 from the other 6 classes.
数据划分#
在这里,我们选择5,000个样本用于训练,10,000个样本用于测试。为了实际重现Tensor Sketch原始论文中的结果,请选择100,000个样本用于训练。
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(
X, y, train_size=5_000, test_size=10_000, random_state=42
)
特征归一化#
现在将特征缩放至[0, 1]范围,以匹配LIBSVM网页中数据集的格式,然后按照Tensor Sketch原始论文[1]中的方法将其归一化到单位长度。
from sklearn.pipeline import make_pipeline
from sklearn.preprocessing import MinMaxScaler, Normalizer
mm = make_pipeline(MinMaxScaler(), Normalizer())
X_train = mm.fit_transform(X_train)
X_test = mm.transform(X_test)
建立基线模型#
作为基线,我们在原始特征上训练一个线性SVM,并打印精度。我们还测量和存储精度和训练时间,以便稍后绘制它们。
import time
from sklearn.svm import LinearSVC
results = {}
lsvm = LinearSVC()
start = time.time()
lsvm.fit(X_train, y_train)
lsvm_time = time.time() - start
lsvm_score = 100 * lsvm.score(X_test, y_test)
results["LSVM"] = {"time": lsvm_time, "score": lsvm_score}
print(f"Linear SVM score on raw features: {lsvm_score:.2f}%")
Linear SVM score on raw features: 75.62%
建立核近似模型#
然后,我们使用PolynomialCountSketch
和不同的n_components
值在生成的特征上训练线性SVM,这表明这些核特征近似值提高了线性分类的精度。在典型的应用场景中,为了相对于线性分类获得改进,n_components
应该大于输入表示中的特征数量。根据经验,评估分数/运行时间成本的最佳值通常在n_components
= 10 * n_features
附近实现,但这可能取决于正在处理的特定数据集。请注意,由于原始样本具有54个特征,因此四次多项式核的显式特征映射将具有大约850万个特征(精确地说,是54^4)。借助PolynomialCountSketch
,我们可以将该特征空间的大部分判别信息压缩成更紧凑的表示。虽然在这个例子中我们只运行一次实验(n_runs
= 1),但在实践中,应该重复多次实验以弥补PolynomialCountSketch
的随机性。
from sklearn.kernel_approximation import PolynomialCountSketch
n_runs = 1
N_COMPONENTS = [250, 500, 1000, 2000]
for n_components in N_COMPONENTS:
ps_lsvm_time = 0
ps_lsvm_score = 0
for _ in range(n_runs):
pipeline = make_pipeline(
PolynomialCountSketch(n_components=n_components, degree=4),
LinearSVC(),
)
start = time.time()
pipeline.fit(X_train, y_train)
ps_lsvm_time += time.time() - start
ps_lsvm_score += 100 * pipeline.score(X_test, y_test)
ps_lsvm_time /= n_runs
ps_lsvm_score /= n_runs
results[f"LSVM + PS({n_components})"] = {
"time": ps_lsvm_time,
"score": ps_lsvm_score,
}
print(
f"Linear SVM score on {n_components} PolynomialCountSketch "
+ f"features: {ps_lsvm_score:.2f}%"
)
Linear SVM score on 250 PolynomialCountSketch features: 76.55%
Linear SVM score on 500 PolynomialCountSketch features: 76.92%
Linear SVM score on 1000 PolynomialCountSketch features: 77.79%
Linear SVM score on 2000 PolynomialCountSketch features: 78.59%
建立核化SVM模型#
训练一个核化SVM,看看PolynomialCountSketch
对核性能的逼近程度如何。当然,这可能需要一些时间,因为SVC类的可扩展性相对较差。这就是核近似器如此有用的原因。
from sklearn.svm import SVC
ksvm = SVC(C=500.0, kernel="poly", degree=4, coef0=0, gamma=1.0)
start = time.time()
ksvm.fit(X_train, y_train)
ksvm_time = time.time() - start
ksvm_score = 100 * ksvm.score(X_test, y_test)
results["KSVM"] = {"time": ksvm_time, "score": ksvm_score}
print(f"Kernel-SVM score on raw features: {ksvm_score:.2f}%")
Kernel-SVM score on raw features: 79.78%
比较结果#
最后,将不同方法的结果与其训练时间作图。我们可以看到,核化SVM达到了更高的精度,但其训练时间长得多,更重要的是,如果训练样本数量增加,其训练时间将增长得更快。
import matplotlib.pyplot as plt
fig, ax = plt.subplots(figsize=(7, 7))
ax.scatter(
[
results["LSVM"]["time"],
],
[
results["LSVM"]["score"],
],
label="Linear SVM",
c="green",
marker="^",
)
ax.scatter(
[
results["LSVM + PS(250)"]["time"],
],
[
results["LSVM + PS(250)"]["score"],
],
label="Linear SVM + PolynomialCountSketch",
c="blue",
)
for n_components in N_COMPONENTS:
ax.scatter(
[
results[f"LSVM + PS({n_components})"]["time"],
],
[
results[f"LSVM + PS({n_components})"]["score"],
],
c="blue",
)
ax.annotate(
f"n_comp.={n_components}",
(
results[f"LSVM + PS({n_components})"]["time"],
results[f"LSVM + PS({n_components})"]["score"],
),
xytext=(-30, 10),
textcoords="offset pixels",
)
ax.scatter(
[
results["KSVM"]["time"],
],
[
results["KSVM"]["score"],
],
label="Kernel SVM",
c="red",
marker="x",
)
ax.set_xlabel("Training time (s)")
ax.set_ylabel("Accuracy (%)")
ax.legend()
plt.show()
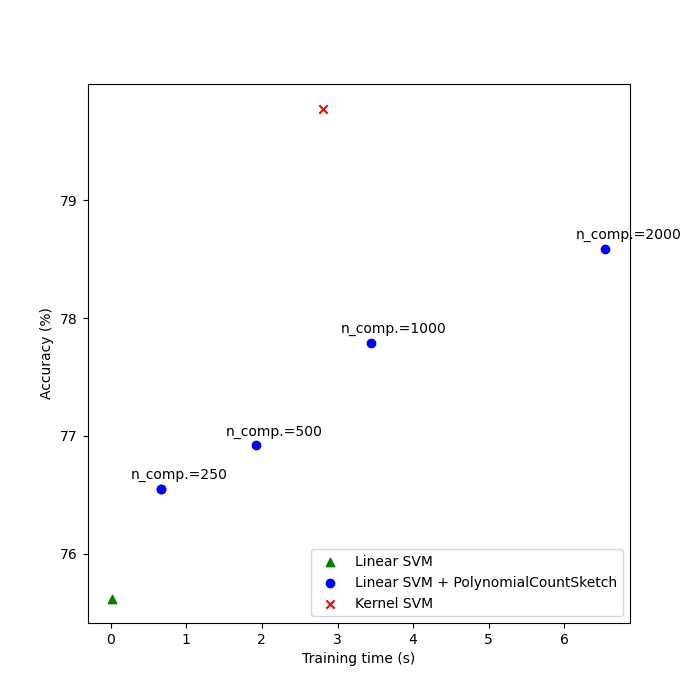
参考文献#
[1] Pham, Ninh and Rasmus Pagh。“通过显式特征映射实现快速且可扩展的多项式核。”KDD '13 (2013)。 https://doi.org/10.1145/2487575.2487591
[2] LIBSVM二元数据集存储库 https://www.csie.ntu.edu.tw/~cjlin/libsvmtools/datasets/binary.html
脚本总运行时间:(0分钟23.161秒)
相关示例