注意
跳转到结尾 下载完整的示例代码。或者通过JupyterLite或Binder在浏览器中运行此示例
人脸数据集分解#
此示例应用于Olivetti人脸数据集,使用来自模块sklearn.decomposition
的不同无监督矩阵分解(降维)方法(参见文档章节将信号分解为成分(矩阵分解问题))。
作者:Vlad Niculae,Alexandre Gramfort
许可证:BSD 3条款
# Authors: The scikit-learn developers
# SPDX-License-Identifier: BSD-3-Clause
数据集准备#
加载和预处理Olivetti人脸数据集。
import logging
import matplotlib.pyplot as plt
from numpy.random import RandomState
from sklearn import cluster, decomposition
from sklearn.datasets import fetch_olivetti_faces
rng = RandomState(0)
# Display progress logs on stdout
logging.basicConfig(level=logging.INFO, format="%(asctime)s %(levelname)s %(message)s")
faces, _ = fetch_olivetti_faces(return_X_y=True, shuffle=True, random_state=rng)
n_samples, n_features = faces.shape
# Global centering (focus on one feature, centering all samples)
faces_centered = faces - faces.mean(axis=0)
# Local centering (focus on one sample, centering all features)
faces_centered -= faces_centered.mean(axis=1).reshape(n_samples, -1)
print("Dataset consists of %d faces" % n_samples)
Dataset consists of 400 faces
定义一个用于绘制人脸图库的基本函数。
n_row, n_col = 2, 3
n_components = n_row * n_col
image_shape = (64, 64)
def plot_gallery(title, images, n_col=n_col, n_row=n_row, cmap=plt.cm.gray):
fig, axs = plt.subplots(
nrows=n_row,
ncols=n_col,
figsize=(2.0 * n_col, 2.3 * n_row),
facecolor="white",
constrained_layout=True,
)
fig.set_constrained_layout_pads(w_pad=0.01, h_pad=0.02, hspace=0, wspace=0)
fig.set_edgecolor("black")
fig.suptitle(title, size=16)
for ax, vec in zip(axs.flat, images):
vmax = max(vec.max(), -vec.min())
im = ax.imshow(
vec.reshape(image_shape),
cmap=cmap,
interpolation="nearest",
vmin=-vmax,
vmax=vmax,
)
ax.axis("off")
fig.colorbar(im, ax=axs, orientation="horizontal", shrink=0.99, aspect=40, pad=0.01)
plt.show()
让我们来看一下我们的数据。灰色表示负值,白色表示正值。
plot_gallery("Faces from dataset", faces_centered[:n_components])
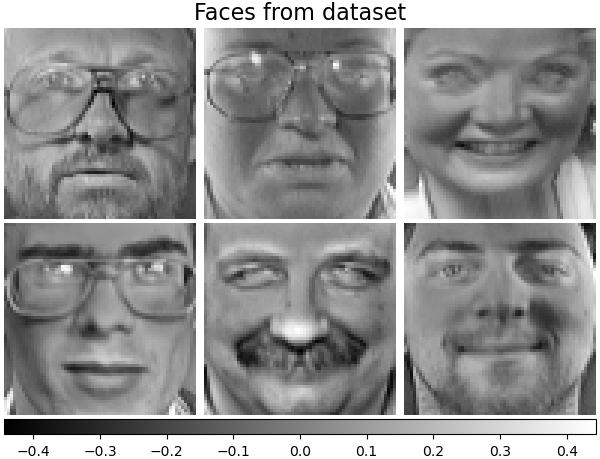
分解#
初始化不同的分解估计器,并对所有图像进行拟合,然后绘制一些结果。每个估计器提取6个成分作为向量\(h \in \mathbb{R}^{4096}\)。我们只是将这些向量以用户友好的可视化方式显示为64x64像素的图像。
在用户指南中了解更多信息。
特征脸 - 使用随机SVD的PCA#
使用数据的奇异值分解 (SVD) 进行线性降维,以将其投影到低维空间。
注意
Eigenfaces估计器,通过sklearn.decomposition.PCA
,还提供了一个标量noise_variance_
(像素方差的均值),它不能显示为图像。
pca_estimator = decomposition.PCA(
n_components=n_components, svd_solver="randomized", whiten=True
)
pca_estimator.fit(faces_centered)
plot_gallery(
"Eigenfaces - PCA using randomized SVD", pca_estimator.components_[:n_components]
)
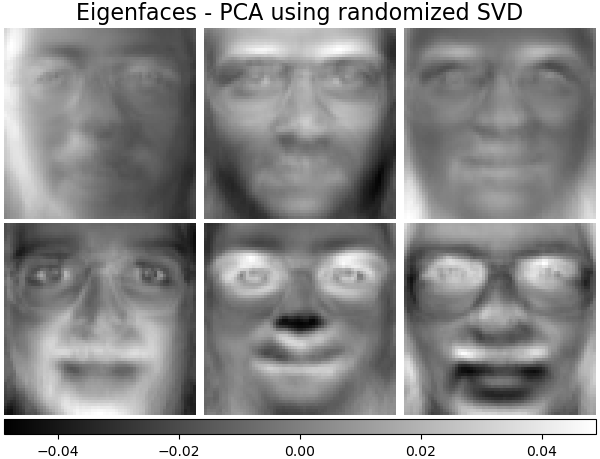
非负成分 - NMF#
将非负原始数据估计为两个非负矩阵的乘积。
nmf_estimator = decomposition.NMF(n_components=n_components, tol=5e-3)
nmf_estimator.fit(faces) # original non- negative dataset
plot_gallery("Non-negative components - NMF", nmf_estimator.components_[:n_components])
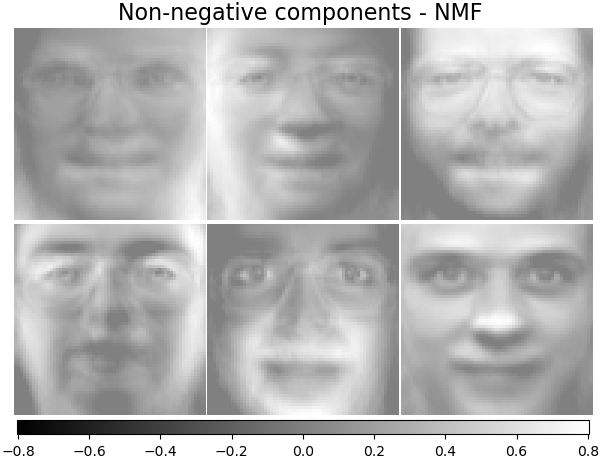
独立成分 - FastICA#
独立成分分析将多元向量分离成最大独立的加性子成分。
ica_estimator = decomposition.FastICA(
n_components=n_components, max_iter=400, whiten="arbitrary-variance", tol=15e-5
)
ica_estimator.fit(faces_centered)
plot_gallery(
"Independent components - FastICA", ica_estimator.components_[:n_components]
)
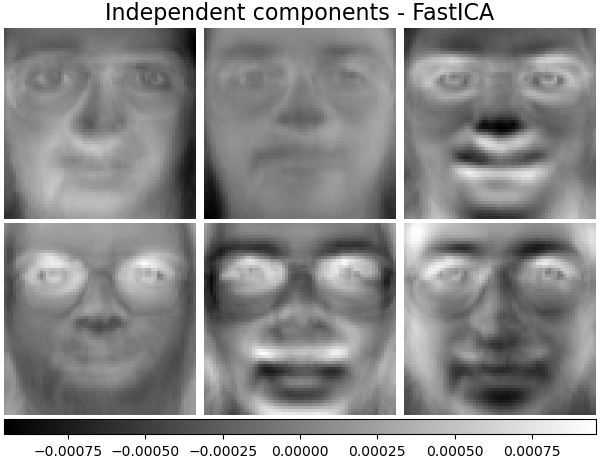
稀疏成分 - MiniBatchSparsePCA#
Mini-batch稀疏PCA (MiniBatchSparsePCA
) 提取最能重构数据的稀疏成分集。此变体比类似的SparsePCA
更快但精度较低。
batch_pca_estimator = decomposition.MiniBatchSparsePCA(
n_components=n_components, alpha=0.1, max_iter=100, batch_size=3, random_state=rng
)
batch_pca_estimator.fit(faces_centered)
plot_gallery(
"Sparse components - MiniBatchSparsePCA",
batch_pca_estimator.components_[:n_components],
)
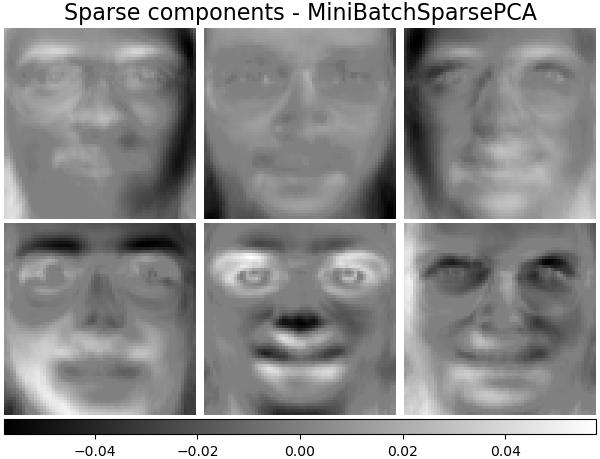
字典学习#
默认情况下,MiniBatchDictionaryLearning
将数据分成小批量,并通过循环遍历指定迭代次数的小批量以在线方式进行优化。
batch_dict_estimator = decomposition.MiniBatchDictionaryLearning(
n_components=n_components, alpha=0.1, max_iter=50, batch_size=3, random_state=rng
)
batch_dict_estimator.fit(faces_centered)
plot_gallery("Dictionary learning", batch_dict_estimator.components_[:n_components])
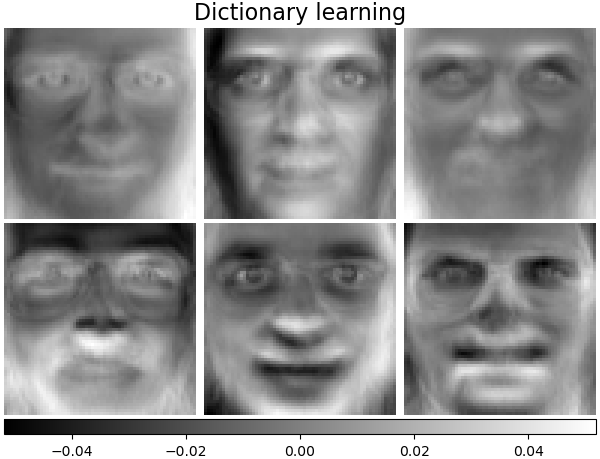
聚类中心 - MiniBatchKMeans#
sklearn.cluster.MiniBatchKMeans
计算效率高,并使用partial_fit
方法实现在线学习。这就是为什么使用MiniBatchKMeans
来增强一些耗时的算法可能是有益的。
kmeans_estimator = cluster.MiniBatchKMeans(
n_clusters=n_components,
tol=1e-3,
batch_size=20,
max_iter=50,
random_state=rng,
)
kmeans_estimator.fit(faces_centered)
plot_gallery(
"Cluster centers - MiniBatchKMeans",
kmeans_estimator.cluster_centers_[:n_components],
)
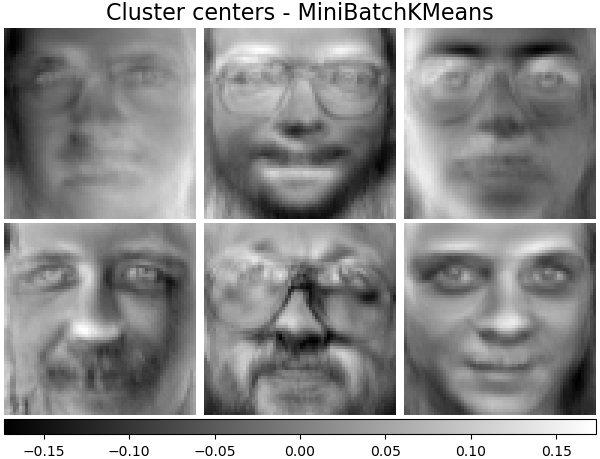
因子分析成分 - FA#
FactorAnalysis
类似于PCA
,但它具有独立建模输入空间每个方向的方差(异方差噪声)的优势。在用户指南中了解更多信息。
fa_estimator = decomposition.FactorAnalysis(n_components=n_components, max_iter=20)
fa_estimator.fit(faces_centered)
plot_gallery("Factor Analysis (FA)", fa_estimator.components_[:n_components])
# --- Pixelwise variance
plt.figure(figsize=(3.2, 3.6), facecolor="white", tight_layout=True)
vec = fa_estimator.noise_variance_
vmax = max(vec.max(), -vec.min())
plt.imshow(
vec.reshape(image_shape),
cmap=plt.cm.gray,
interpolation="nearest",
vmin=-vmax,
vmax=vmax,
)
plt.axis("off")
plt.title("Pixelwise variance from \n Factor Analysis (FA)", size=16, wrap=True)
plt.colorbar(orientation="horizontal", shrink=0.8, pad=0.03)
plt.show()
分解:字典学习#
在接下来的部分中,让我们更精确地考虑字典学习。字典学习是一个问题,它相当于找到输入数据的稀疏表示作为简单元素组合。这些简单元素构成一个字典。可以将字典和/或编码系数限制为正值,以匹配数据中可能存在的约束。
MiniBatchDictionaryLearning
实现了一个更快但精度较低的字典学习算法版本,更适合大型数据集。在用户指南中了解更多信息。
绘制数据集中的相同样本,但使用另一个颜色映射。红色表示负值,蓝色表示正值,白色表示零。
plot_gallery("Faces from dataset", faces_centered[:n_components], cmap=plt.cm.RdBu)
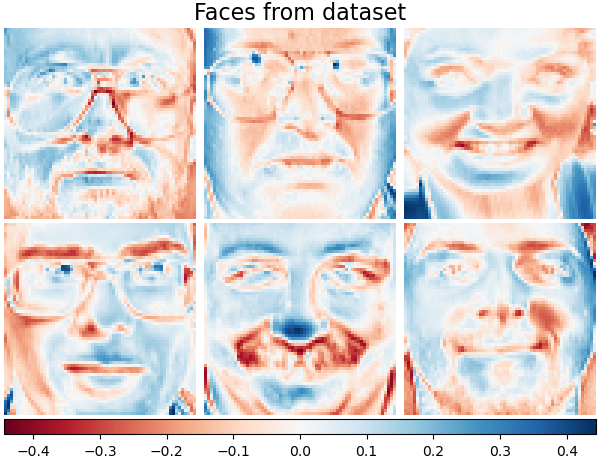
与之前的示例类似,我们更改参数并训练MiniBatchDictionaryLearning
估计器对所有图像进行处理。通常,字典学习和稀疏编码将输入数据分解为字典和编码系数矩阵。\(X \approx UV\),其中\(X = [x_1, . . . , x_n]\),\(X \in \mathbb{R}^{m×n}\),字典\(U \in \mathbb{R}^{m×k}\),编码系数\(V \in \mathbb{R}^{k×n}\)。
以下是字典和编码系数都施加正约束时的结果。
字典学习 - 正字典#
在以下部分,我们将在寻找字典时强制执行正值约束。
dict_pos_dict_estimator = decomposition.MiniBatchDictionaryLearning(
n_components=n_components,
alpha=0.1,
max_iter=50,
batch_size=3,
random_state=rng,
positive_dict=True,
)
dict_pos_dict_estimator.fit(faces_centered)
plot_gallery(
"Dictionary learning - positive dictionary",
dict_pos_dict_estimator.components_[:n_components],
cmap=plt.cm.RdBu,
)
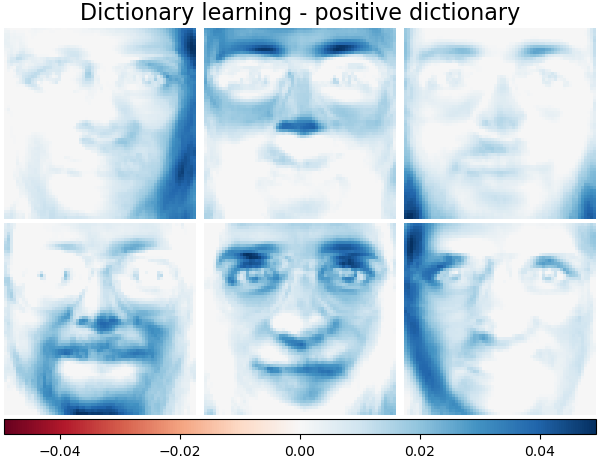
字典学习 - 正编码#
在下文中,我们将编码系数约束为正矩阵。
dict_pos_code_estimator = decomposition.MiniBatchDictionaryLearning(
n_components=n_components,
alpha=0.1,
max_iter=50,
batch_size=3,
fit_algorithm="cd",
random_state=rng,
positive_code=True,
)
dict_pos_code_estimator.fit(faces_centered)
plot_gallery(
"Dictionary learning - positive code",
dict_pos_code_estimator.components_[:n_components],
cmap=plt.cm.RdBu,
)
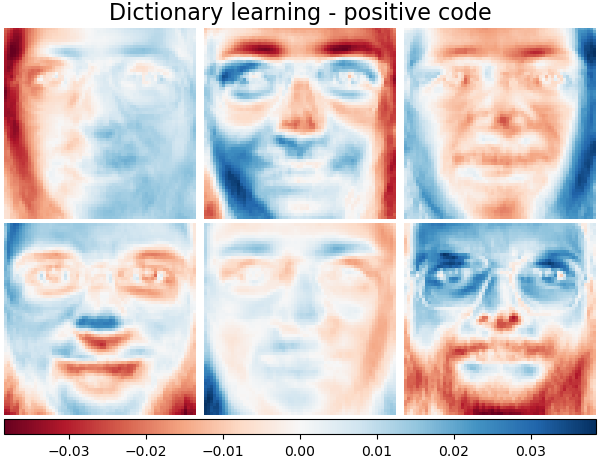
字典学习 - 正字典 & 正编码#
以下是字典值和编码系数都施加正约束时的结果。
dict_pos_estimator = decomposition.MiniBatchDictionaryLearning(
n_components=n_components,
alpha=0.1,
max_iter=50,
batch_size=3,
fit_algorithm="cd",
random_state=rng,
positive_dict=True,
positive_code=True,
)
dict_pos_estimator.fit(faces_centered)
plot_gallery(
"Dictionary learning - positive dictionary & code",
dict_pos_estimator.components_[:n_components],
cmap=plt.cm.RdBu,
)
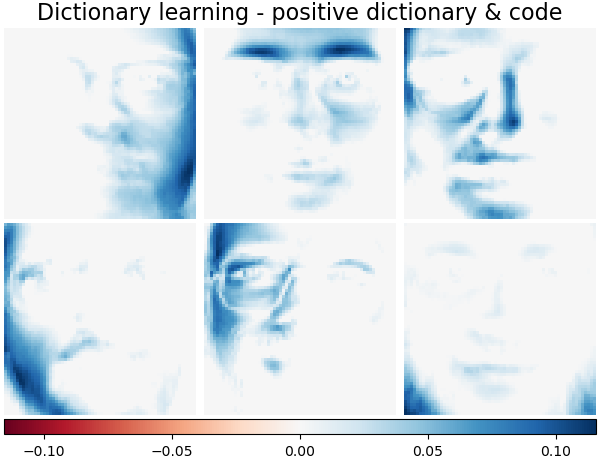
脚本总运行时间:(0 分钟 8.157 秒)
相关示例