注意
转到末尾 下载完整的示例代码。或者通过JupyterLite或Binder在浏览器中运行此示例
单个估计器与Bagging:偏差-方差分解#
此示例说明并比较了单个估计器与Bagging集成方法的预期均方误差的偏差-方差分解。
在回归分析中,估计量的期望均方误差可以分解为偏差、方差和噪声三部分。在回归问题的各个数据集上的平均值上,偏差项衡量的是估计器的预测结果与该问题最佳估计器(即贝叶斯模型)预测结果之间的平均差异。方差项衡量的是在同一问题的不同随机实例上拟合估计器时,其预测结果的变异性。以下将每个问题实例记为“LS”(“学习样本”)。最后,噪声衡量的是由于数据变异性造成的不可约误差部分。
左上图展示了在一个玩具一维回归问题的随机数据集 LS(蓝点)上训练的单个决策树的预测结果(深红色)。它还展示了在该问题的其他(不同)随机抽取的 LS 实例上训练的其他单个决策树的预测结果(浅红色)。直观地说,这里的方差项对应于单个估计器预测结果(浅红色)的宽度。方差越大,预测结果对x
的训练集的小变化越敏感。偏差项对应于估计器的平均预测结果(青色)与最佳模型(深蓝色)之间的差异。在这个问题上,我们可以观察到偏差相当低(青色和蓝色曲线彼此接近),而方差很大(红色光束相当宽)。
左下图绘制了单个决策树的期望均方误差的逐点分解。它证实了偏差项(蓝色)较低,而方差项(绿色)较大。它还展示了误差的噪声部分,正如预期的那样,它似乎是常数,约为0.01
。
右边的图对应于相同的绘图,但使用的是决策树的 Bagging 集成。在这两幅图中,我们可以观察到偏差项比前面情况更大。在右上图中,平均预测结果(青色)与最佳模型之间的差异更大(例如,注意x=2
附近的偏移)。在右下图中,偏差曲线也略高于左下图。然而,就方差而言,预测结果的光束更窄,这表明方差更低。事实上,正如右下图所示,方差项(绿色)比单个决策树的方差项更低。总的来说,偏差-方差分解不再相同。Bagging 的权衡更好:对数据集的 bootstrap 副本拟合多个决策树的平均值会稍微增加偏差项,但可以更大程度地减少方差,从而导致总体均方误差更低(比较下图中的红色曲线)。脚本输出也证实了这种直觉。Bagging 集成的总误差低于单个决策树的总误差,而这种差异实际上主要源于方差的减少。
有关偏差-方差分解的更多详细信息,请参见[1]的 7.3 节。
参考文献#
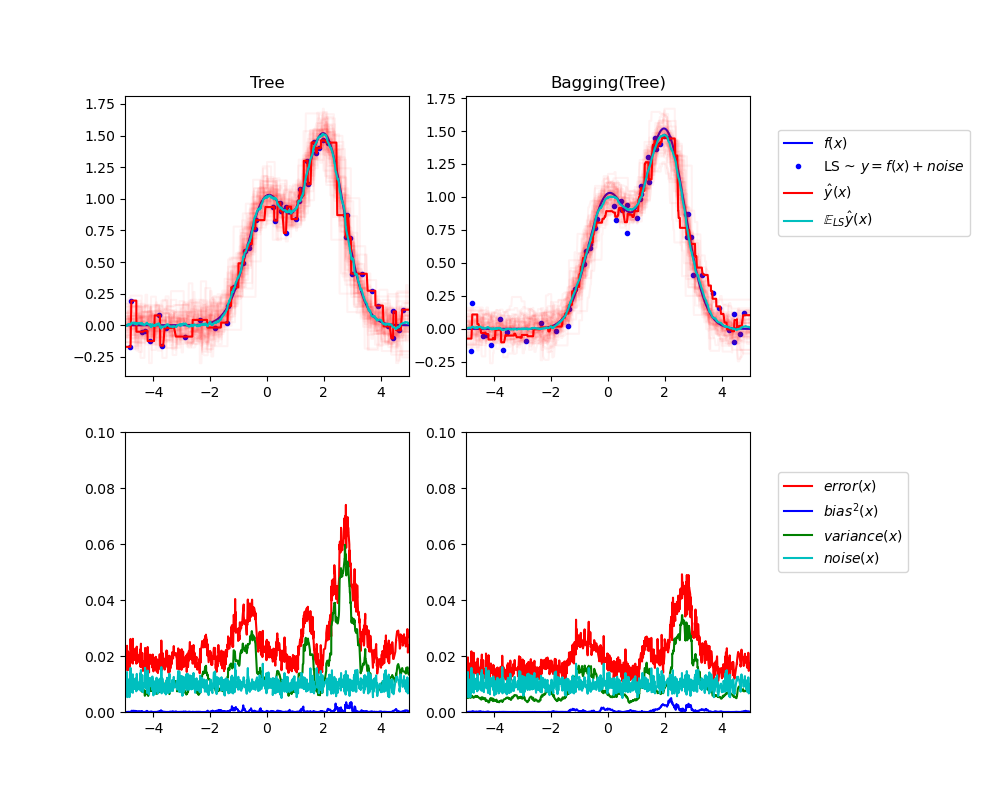
Tree: 0.0255 (error) = 0.0003 (bias^2) + 0.0152 (var) + 0.0098 (noise)
Bagging(Tree): 0.0196 (error) = 0.0004 (bias^2) + 0.0092 (var) + 0.0098 (noise)
# Authors: The scikit-learn developers
# SPDX-License-Identifier: BSD-3-Clause
import matplotlib.pyplot as plt
import numpy as np
from sklearn.ensemble import BaggingRegressor
from sklearn.tree import DecisionTreeRegressor
# Settings
n_repeat = 50 # Number of iterations for computing expectations
n_train = 50 # Size of the training set
n_test = 1000 # Size of the test set
noise = 0.1 # Standard deviation of the noise
np.random.seed(0)
# Change this for exploring the bias-variance decomposition of other
# estimators. This should work well for estimators with high variance (e.g.,
# decision trees or KNN), but poorly for estimators with low variance (e.g.,
# linear models).
estimators = [
("Tree", DecisionTreeRegressor()),
("Bagging(Tree)", BaggingRegressor(DecisionTreeRegressor())),
]
n_estimators = len(estimators)
# Generate data
def f(x):
x = x.ravel()
return np.exp(-(x**2)) + 1.5 * np.exp(-((x - 2) ** 2))
def generate(n_samples, noise, n_repeat=1):
X = np.random.rand(n_samples) * 10 - 5
X = np.sort(X)
if n_repeat == 1:
y = f(X) + np.random.normal(0.0, noise, n_samples)
else:
y = np.zeros((n_samples, n_repeat))
for i in range(n_repeat):
y[:, i] = f(X) + np.random.normal(0.0, noise, n_samples)
X = X.reshape((n_samples, 1))
return X, y
X_train = []
y_train = []
for i in range(n_repeat):
X, y = generate(n_samples=n_train, noise=noise)
X_train.append(X)
y_train.append(y)
X_test, y_test = generate(n_samples=n_test, noise=noise, n_repeat=n_repeat)
plt.figure(figsize=(10, 8))
# Loop over estimators to compare
for n, (name, estimator) in enumerate(estimators):
# Compute predictions
y_predict = np.zeros((n_test, n_repeat))
for i in range(n_repeat):
estimator.fit(X_train[i], y_train[i])
y_predict[:, i] = estimator.predict(X_test)
# Bias^2 + Variance + Noise decomposition of the mean squared error
y_error = np.zeros(n_test)
for i in range(n_repeat):
for j in range(n_repeat):
y_error += (y_test[:, j] - y_predict[:, i]) ** 2
y_error /= n_repeat * n_repeat
y_noise = np.var(y_test, axis=1)
y_bias = (f(X_test) - np.mean(y_predict, axis=1)) ** 2
y_var = np.var(y_predict, axis=1)
print(
"{0}: {1:.4f} (error) = {2:.4f} (bias^2) "
" + {3:.4f} (var) + {4:.4f} (noise)".format(
name, np.mean(y_error), np.mean(y_bias), np.mean(y_var), np.mean(y_noise)
)
)
# Plot figures
plt.subplot(2, n_estimators, n + 1)
plt.plot(X_test, f(X_test), "b", label="$f(x)$")
plt.plot(X_train[0], y_train[0], ".b", label="LS ~ $y = f(x)+noise$")
for i in range(n_repeat):
if i == 0:
plt.plot(X_test, y_predict[:, i], "r", label=r"$\^y(x)$")
else:
plt.plot(X_test, y_predict[:, i], "r", alpha=0.05)
plt.plot(X_test, np.mean(y_predict, axis=1), "c", label=r"$\mathbb{E}_{LS} \^y(x)$")
plt.xlim([-5, 5])
plt.title(name)
if n == n_estimators - 1:
plt.legend(loc=(1.1, 0.5))
plt.subplot(2, n_estimators, n_estimators + n + 1)
plt.plot(X_test, y_error, "r", label="$error(x)$")
plt.plot(X_test, y_bias, "b", label="$bias^2(x)$"),
plt.plot(X_test, y_var, "g", label="$variance(x)$"),
plt.plot(X_test, y_noise, "c", label="$noise(x)$")
plt.xlim([-5, 5])
plt.ylim([0, 0.1])
if n == n_estimators - 1:
plt.legend(loc=(1.1, 0.5))
plt.subplots_adjust(right=0.75)
plt.show()
脚本总运行时间:(0 分钟 1.428 秒)
相关示例