注意
转到末尾下载完整示例代码。或通过 JupyterLite 或 Binder 在浏览器中运行此示例
欠拟合与过拟合#
本示例演示了欠拟合和过拟合的问题,以及我们如何使用带多项式特征的线性回归来近似非线性函数。图中显示了我们希望近似的函数,它是余弦函数的一部分。此外,还展示了真实函数样本和不同模型的近似。这些模型具有不同次数的多项式特征。我们可以看到,线性函数(1 次多项式)不足以拟合训练样本。这称为**欠拟合**。4 次多项式可以几乎完美地近似真实函数。然而,对于更高次数的模型,它将**过拟合**训练数据,即它学习了训练数据的噪声。我们通过交叉验证定量评估**过拟合**/**欠拟合**。我们计算验证集上的均方误差 (MSE),均方误差越高,模型从训练数据中正确泛化的可能性越低。
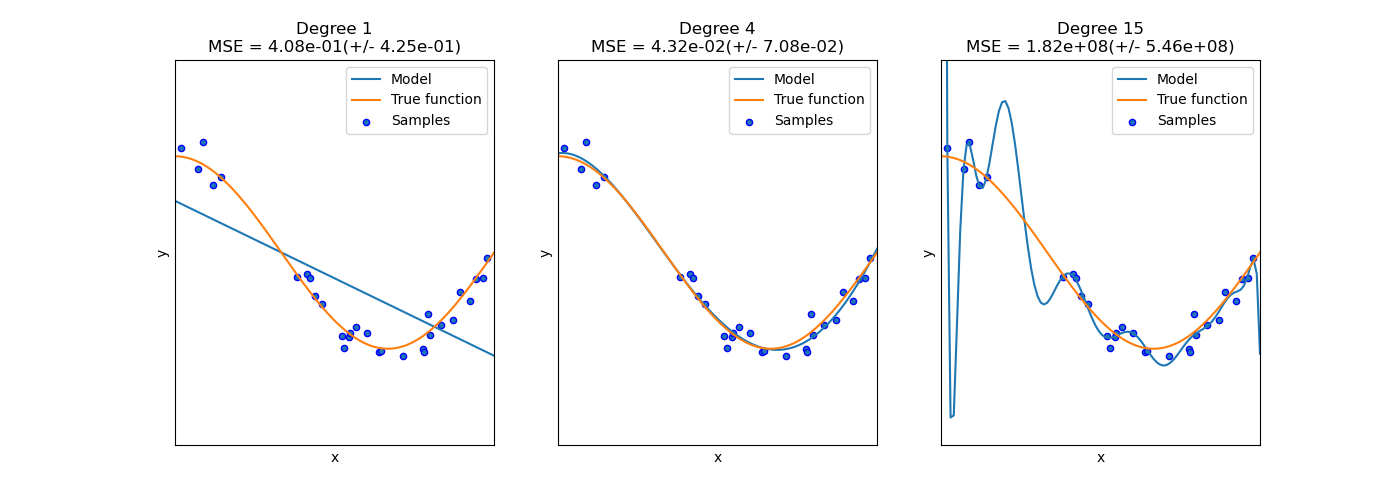
# Authors: The scikit-learn developers
# SPDX-License-Identifier: BSD-3-Clause
import matplotlib.pyplot as plt
import numpy as np
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import cross_val_score
from sklearn.pipeline import Pipeline
from sklearn.preprocessing import PolynomialFeatures
def true_fun(X):
return np.cos(1.5 * np.pi * X)
np.random.seed(0)
n_samples = 30
degrees = [1, 4, 15]
X = np.sort(np.random.rand(n_samples))
y = true_fun(X) + np.random.randn(n_samples) * 0.1
plt.figure(figsize=(14, 5))
for i in range(len(degrees)):
ax = plt.subplot(1, len(degrees), i + 1)
plt.setp(ax, xticks=(), yticks=())
polynomial_features = PolynomialFeatures(degree=degrees[i], include_bias=False)
linear_regression = LinearRegression()
pipeline = Pipeline(
[
("polynomial_features", polynomial_features),
("linear_regression", linear_regression),
]
)
pipeline.fit(X[:, np.newaxis], y)
# Evaluate the models using crossvalidation
scores = cross_val_score(
pipeline, X[:, np.newaxis], y, scoring="neg_mean_squared_error", cv=10
)
X_test = np.linspace(0, 1, 100)
plt.plot(X_test, pipeline.predict(X_test[:, np.newaxis]), label="Model")
plt.plot(X_test, true_fun(X_test), label="True function")
plt.scatter(X, y, edgecolor="b", s=20, label="Samples")
plt.xlabel("x")
plt.ylabel("y")
plt.xlim((0, 1))
plt.ylim((-2, 2))
plt.legend(loc="best")
plt.title(
"Degree {}\nMSE = {:.2e}(+/- {:.2e})".format(
degrees[i], -scores.mean(), scores.std()
)
)
plt.show()
脚本总运行时间: (0 分 0.188 秒)
相关示例